Git Cherry-Picking: What It Is and How to Use It
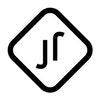
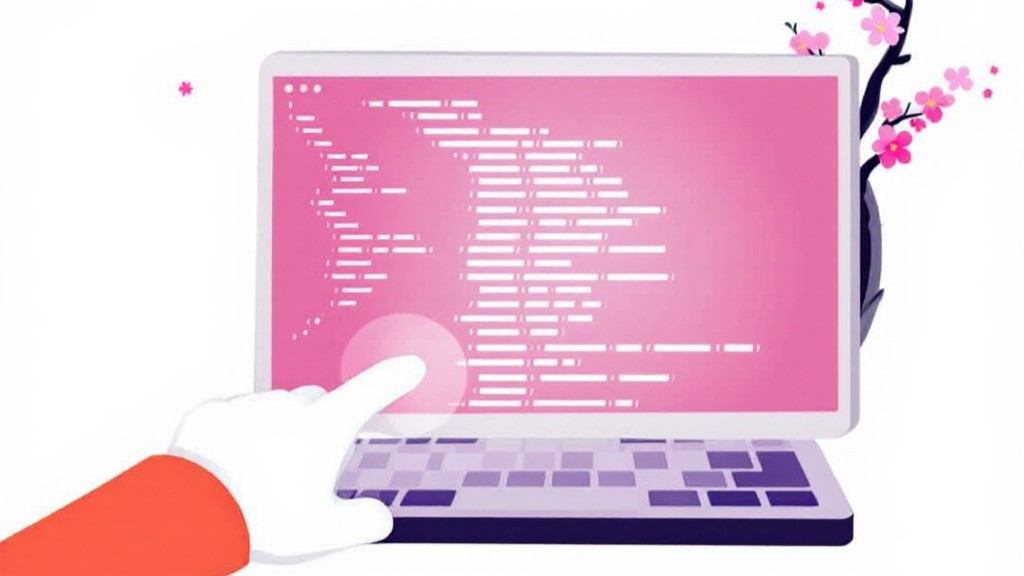
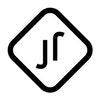
Git is a powerful tool for version control, allowing developers to manage their projects efficiently. One of the many features Git offers is cherry-picking, which allows you to select specific commits from one branch and apply them to another. This is especially useful when you only want to include certain changes from a branch, instead of merging everything.
In this article, we will walk you through what cherry-picking is, how to use it, and why it's an essential tool for developers.
What Is Git Cherry-Picking?
In simple terms, cherry-picking in Git means choosing individual commits from one branch and applying them to another branch. Think of it like picking out a few cherries from a bunch. Instead of merging all the changes from one branch into another, you only bring over the commits you need.
This is incredibly useful in situations where you want to:
- Apply a specific bug fix or feature from another branch.
- Avoid pulling in unwanted changes from a feature branch.
- Backport fixes to an older version of your project without merging everything.
Why Should You Use Cherry-Picking?
- Selective Changes: Cherry-picking allows you to pull in specific changes without affecting the entire codebase.
- Bug Fixes: It's a great way to apply bug fixes from a development branch to a production branch.
- Feature Isolations: If you're working on a feature branch, you can choose only certain commits to be moved to another branch.
Now that we understand why cherry-picking is useful, let's learn how to use it.
How to Cherry-Pick a Commit in Git
Step 1: Find the Commit Hash
Each commit in Git has a unique identifier called a commit hash. To cherry-pick a specific commit, you need to know its hash.
To find the commit hash, open your terminal and run the following command:
git log --oneline
This will display a list of commits, each with a unique hash and a short description of the changes made. Here's an example:
a1b2c3d Fix typo in readme
d4e5f6g Add new feature to dashboard
h7i8j9k Refactor user authentication code
Take note of the commit hash (e.g., a1b2c3d
) of the commit you want to cherry-pick.
Step 2: Switch to the Target Branch
Next, you need to switch to the branch where you want to apply the commit. You can use the following command to do this:
git checkout <target-branch>
For newer versions of Git, you can also use:
git switch <target-branch>
Replace <target-branch>
with the name of the branch where you want to apply the changes (e.g., main
, develop
, or any other branch you're working with).
Step 3: Cherry-Pick the Commit
Once you're on the correct branch, run the following command to cherry-pick the commit:
git cherry-pick <commit-hash>
Replace <commit-hash>
with the hash you noted earlier (e.g., a1b2c3d
).
Git will apply the changes from that commit onto your current branch.
Cherry-Picking Multiple Commits
Sometimes, you may want to cherry-pick multiple commits at once. You can do this by specifying multiple commit hashes.
For example:
git cherry-pick <commit1-hash> <commit2-hash>
You can also cherry-pick a range of commits by using the following syntax:
git cherry-pick <start-commit>^..<end-commit>
The ^
symbol ensures that the start commit is included in the range.
Example:
git cherry-pick a1b2c3d^..d4e5f6g
This will cherry-pick all commits between a1b2c3d
and d4e5f6g
, including both.
Handling Conflicts During Cherry-Picking
When cherry-picking commits, there is a chance that conflicts may arise if the changes from the commit conflict with the current state of the target branch.
How to Resolve Conflicts:
- Git will notify you of any conflicts, and the conflicting files will be marked.
- Open the conflicting files and manually resolve the differences.
- Once you’ve resolved the conflicts, add the changes to the staging area:
git add <resolved-file>
- To continue the cherry-pick operation after resolving conflicts, run:
git cherry-pick --continue
If you decide that you no longer want to cherry-pick the commit (or if you can't resolve the conflicts), you can abort the cherry-pick by using:
git cherry-pick --abort
Cherry-Pick in Real-World Scenarios
Scenario 1: Applying a Bug Fix to Production
Imagine you're working on a development branch, and you've fixed a bug. However, you need to apply that fix to the production branch without bringing in other changes from development. Cherry-picking allows you to apply only the fix to the production branch, keeping everything else in the development branch untouched.
Scenario 2: Backporting Features
Suppose you've added a cool feature to your app in a new release branch, but you also want to add it to an older release version. Instead of merging all the changes from the new branch, you can cherry-pick just the commits related to the new feature and apply them to the older version.
Key Points to Remember
- Cherry-picking is powerful: It gives you control over which commits to apply to a branch.
- Use it carefully: Since cherry-picking only applies individual commits, make sure you're applying the correct changes.
- Conflicts are possible: Be prepared to handle conflicts if the changes in the commit don't mesh well with the current branch.
Git cherry-picking is an incredibly useful feature when you need to apply specific changes from one branch to another. Whether you're fixing bugs, backporting features, or just isolating certain changes, cherry-picking provides a selective way to manage your codebase. By following the steps outlined in this guide, you can confidently use cherry-picking in your development workflow.
Remember, cherry-picking gives you flexibility, but always keep an eye on potential conflicts, and make sure you’re applying the correct commits. With this knowledge, you're ready to use cherry-picking effectively in your Git projects!